The CPI command in Atmega32 with ATMEL STUDIO 7 Assembly stands for “Compare Immediate.” Without changing the register itself, it is utilized to compare a register with an instantaneous value. Depending on the outcome of the comparison, the CPI instruction puts flags in the Status Register. In assembly language programming, this instruction is crucial for conditional branching since it enables decision-making depending on comparison outcomes.
Let us understand this command through a program in assembly language.
Firstly, we will include a header file.
CODE:
.INCLUDE “M32DEF.INC”
If you do not know how to include it, you can check by clicking here.
LDI R16,HIGH(RAMEND)
OUT SPH, R16
LDI R16,LOW(RAMEND)
OUT SPL,R16 // INITIALIZING THE STACK
First, we will initialize the stack.
For initializing the stack, we OUT the High(RAMEND) on the SPH and we OUT the Low(RAMEND) on the SPL. Here SPH means Stack Pointer High and SPL means Stack Pointer Low. It means that we have specified the space for the stack in RAM.
LDI R20,0X00
OUT DDRB,R20 // PORTB CONFIGURED AS input
Then, we loaded the hex value 00 in the general-purpose register R20. After that, we OUT that value on the data direction register of port B. In this way, port B has been configured as input.
IN R20,PINB
As we know that the sensor is connected to PINB, we have to get the data from it, therefore we will use the command IN.
The IN command gets the data from any special function register and gives it to the general-purpose register.
CPI R20,75 // R20=R20-75
BRSH LOOP // EXEXUTES WHEN C=0
CPI command compares the value of the general-purpose register with the immediate value and if the answer is zero it would raise the z flag.
BRSH is a branching command that jumps to the loop or label when we get the 0 carry from the previous statement. And it would jump to the LOOP.
But if the carry is 1 it will not execute this loop infection it will terminate it and execute the following command.
COMMAND
MOV R18,R20 //R18=R20
RJMP LOOP2
The MOV command will simply move the value of the general-purpose register R20 to R18.
LOOP:
BRNE LOOP1
In the loop, we used the command branch not equal. What the BRNE command does is, it remains true until the z flag has the value 0.
But if the z flag has a value of 1, it gets raised, and the BRNE condition becomes false and terminates this command.
MOV R16,R20
RJMP LOOP2
This command will move the value from the general purpose register R20 to a general purpose register R16. And then jumped to LOOP2.
If the statement gets true in BRNE it will jump to loop1 as given below.
LOOP1:
MOV R17,R20
LOOP2:
NOP
JMP LOOP
LOOP1 will simply move the value of the general purpose register R20 to R17.
Now we will build and debug our solution and check the output step by step.
DEBUG:
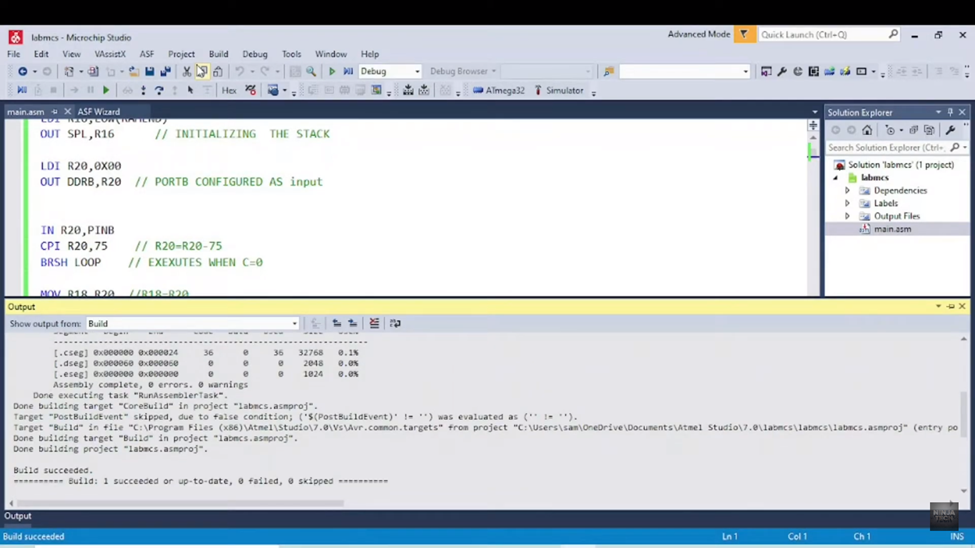
Now we will check our output step by step by pressing the F10 or you can use the option step over from the menu bar.
Firstly, we can see the stack has been initialized.
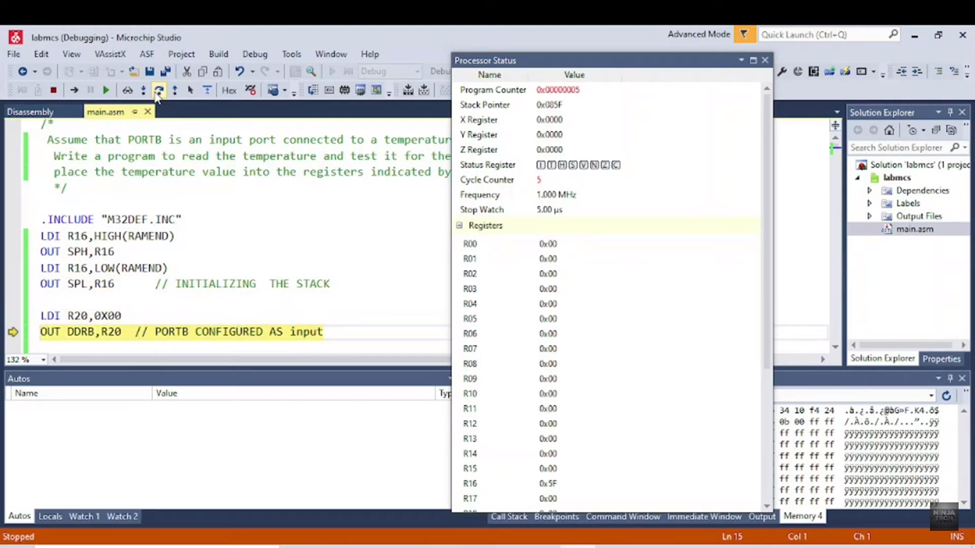
As you can see the carry is 1 which means the BRSH command would become false.
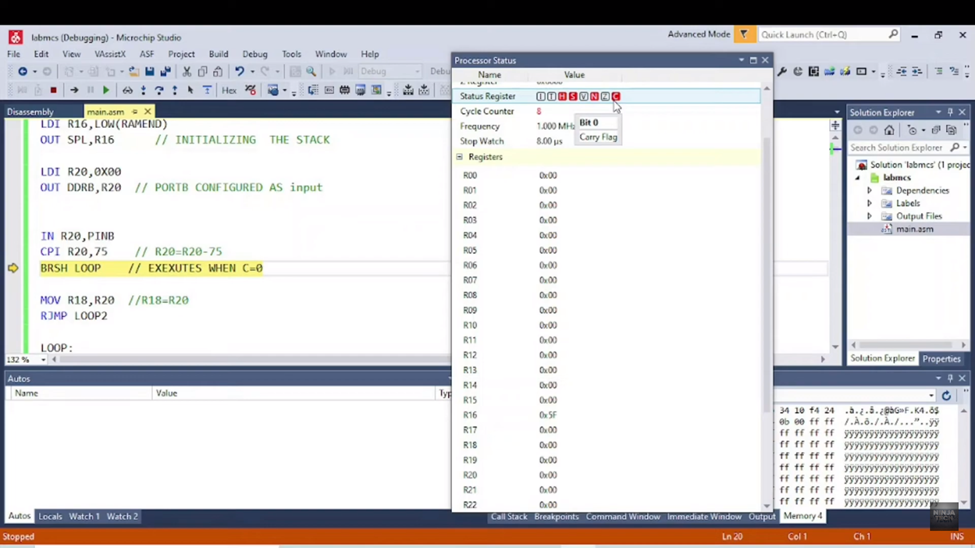
Here, it will move the value from R20 to R18, as the value is 0 it will not be shown.
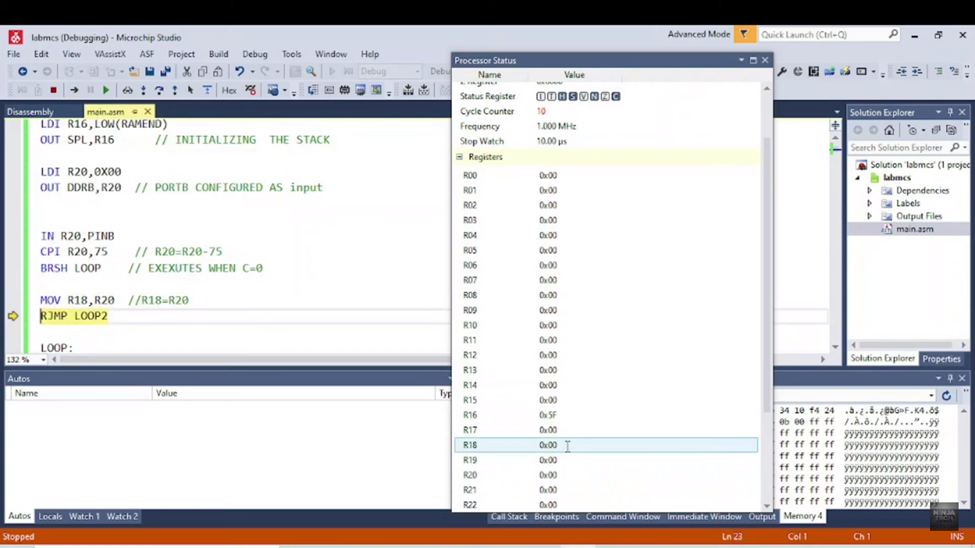
And then the loop continues.
Conclusion
To ascertain equality or inequality, the CPI command in Atmega32 Assembly compares a register with an instantaneous value. Depending on the result of the comparison, it puts flags in the Status Register, including the Zero Flag, Carry Flag, and others. This comparison procedure is essential for providing conditional branching, which allows for the control of program flow by taking alternative pathways based on the comparison result.
For Complete Trial Watch the Video: CPI command in Atmega32 using ATMEL STUDIO 7 Assembly
For more blogs explore the website: https://ninjatech.live/