Unsigned integers (often called “units”) are just like integers (whole numbers) but have the property that they don’t have a positive + or negative – sign associated with them. Thus, they are always non-negative (zero or positive).
Let us understand it through a program in assembly language.
Firstly, we will include a header file.
CODE:
.INCLUDE “M32DEF.INC”
If you do not know how to include it, you can check by clicking here.
.DEF num = R20
.DEF denominator = R21
.DEF Quotient = R22
Above we assigned the variables to general purpose registers like variable num assigned to the general purpose register R20, denominator to R21, and quotient to R22.
LDI num, 95 //R20=95
LDI denominator, 10 // R21=10
CLR Quotient // R22=0
Here we loaded the immediate value 95 in the num variable but on the backend, the num variable has been assigned to R20 so it would be equal to R20.
And the immediate value 10 loaded in the variable denominator. Similarly, on the backend, the variable denominator is equal to R21.
Then we cleared the quotient so that if there is already a value it would be cleared.
L1: INC Quotient // R22=R22+1
SUB num, denominator // R20=R20-R21
BRCC L1 // BRANCH IF C=0
What we did in the first step of the label is we just incremented the value of the quotient. In the next step, we subtracted the value of the denominator from the numerator.
In the next step, BRCC means jump to label L1 if carry is equal to 0. Mean that branch only if the value of the carry is equal to 0.
DEC Quotient // R22=R22-1
ADD num, denominator // R20=R20+R21
Here: JMP Here
But if the carry is not zero then decrement in the value of the quotient and then add the value of the denominator in the value of the numerator. And in this way, the loop goes on.
Now we will build and debug our solution and check the output step by step.
DEBUG:
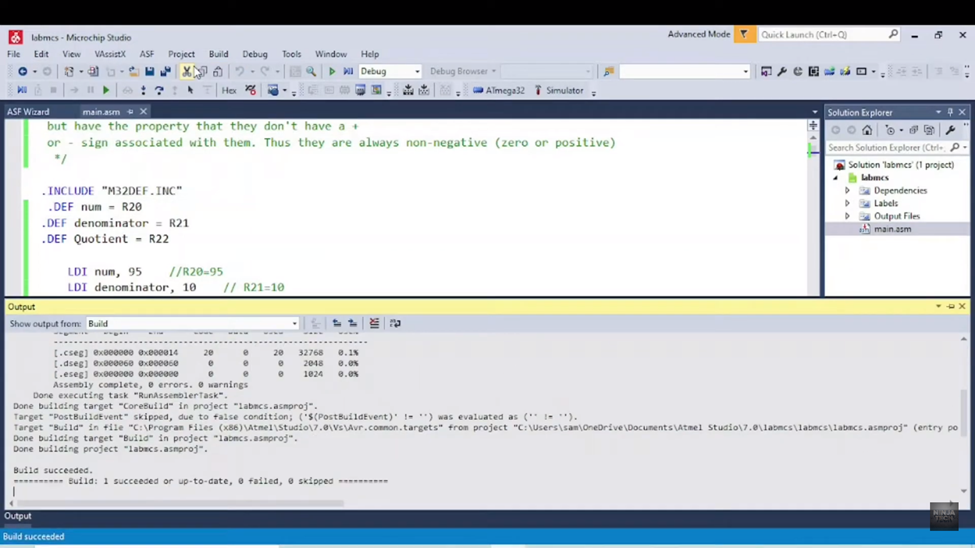
Now we will check our output step by step by pressing the F10 or you can use the option step over from the menu bar.
Below you can see the value of the numerator and denominator has been loaded in the right registers.
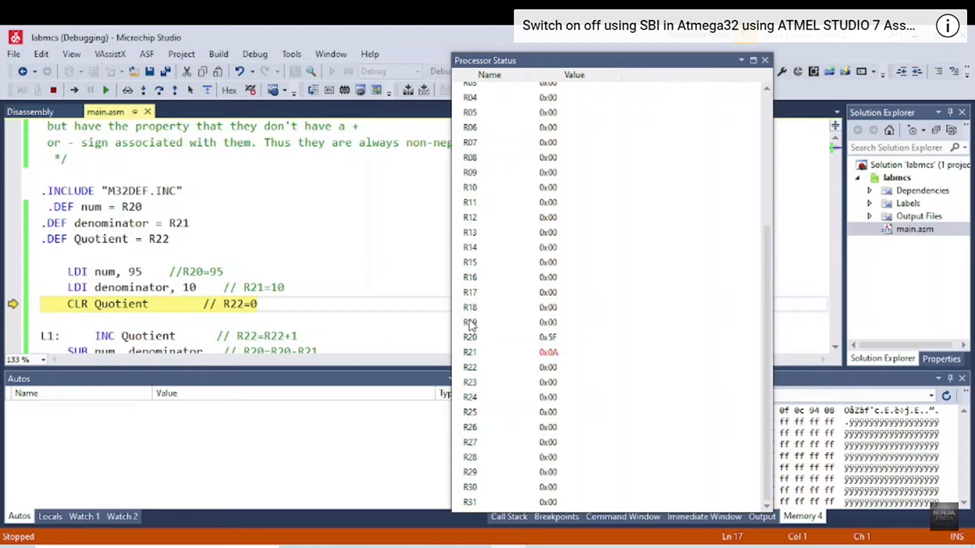
In the next step, you can see that the value has been incremented in the quotient which is R22.
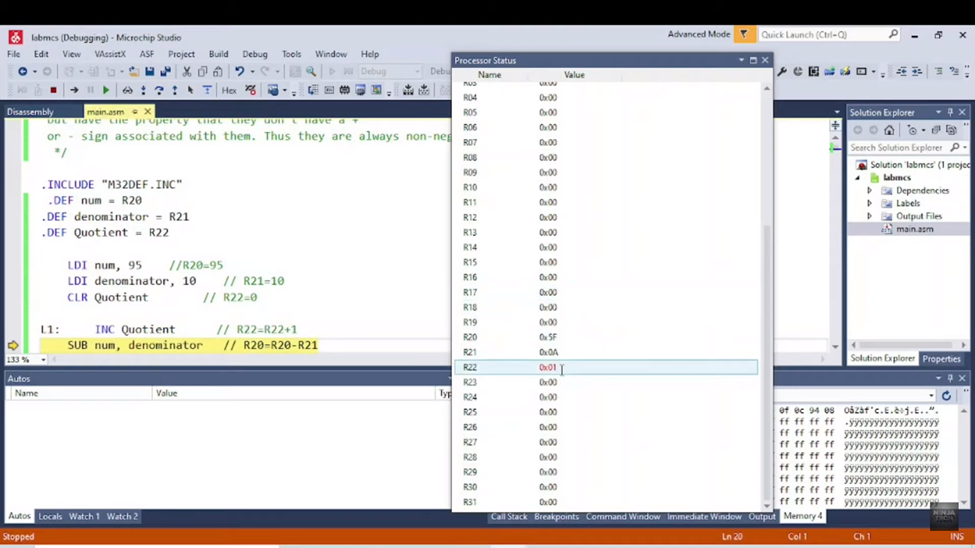
And then we have the subtracted value in the R20 because we have subtracted the denominator from the numerator.
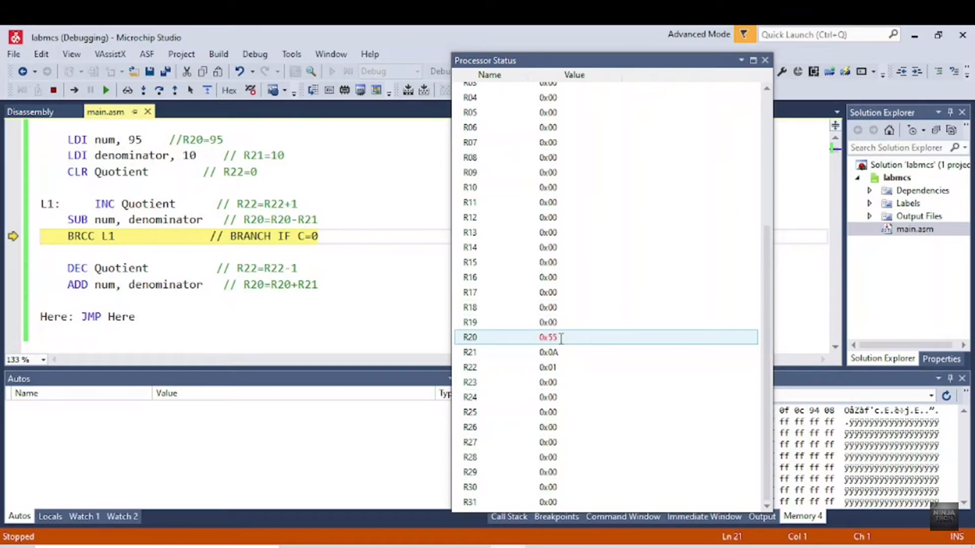
It will continue to jump to label L1 until the carry flag is raised. Below you can see that the carry flag has been raised so now it will terminate it and jump to the next instruction.
Below we have the quotient value 09 and the remaining value in the register R20. And the loop continues.
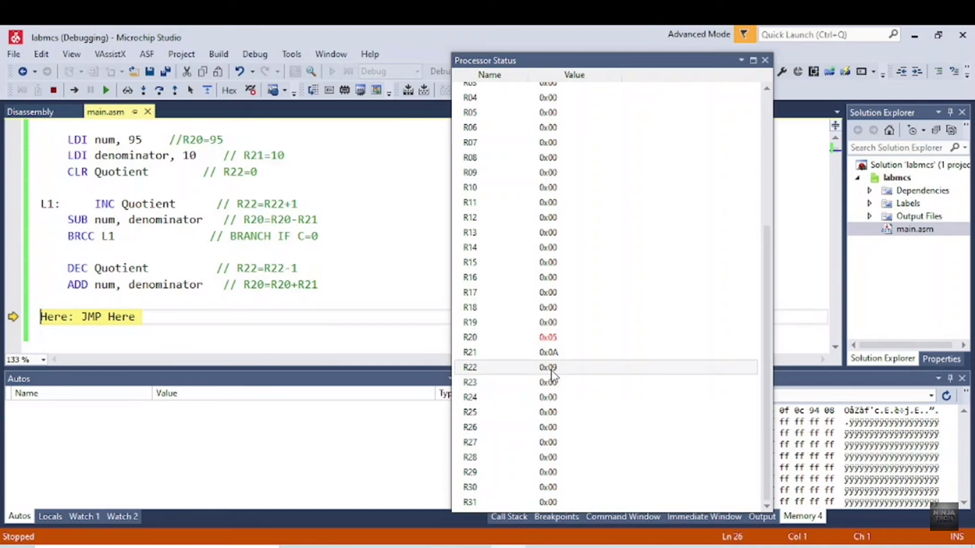
Conclusion
Unsigned division excludes negative values and only works with positive integers. To obtain correct results while handling negative values in the signed division, extra measures must be taken, particularly when dealing with negative dividends or divisors. When working with division operations in the Atmega32 Assembly language, it is important to comprehend these differences.
For Complete Trial Watch the Video: Division in Atmega32 using ATMEL STUDIO 7 Assembly
For more blogs explore the website: https://ninjatech.live/