Here is a solution and code for LED PATTERN in Atmega32 using ATMEL STUDIO. Blinking an LED with a delay is the initial step in programming a microcontroller, much to writing “Hello World” in C or C++. An extremely well-liked, high-performance 8-bit AVR microcontroller is the Atmega32. DDR and PORT are the two registers that must be used for this sample project. The microcontroller’s DDR, or data direction register, sets the input/output direction of each pin.
Corresponding pin outputs are made when the DDR register is HIGH, and corresponding pin inputs are made when the DDR register is LOW. The output register that ascertains the condition of every pin on a certain port is called the PORT register. The corresponding pin logic high (5V) is made when the PORT register is HIGH, and the corresponding pin logic low (0V) is made when the PORT register is LOW.
Let us understand it through a program in assembly language.
Firstly, we will include a header file.
CODE:
.INCLUDE “M32DEF.INC”
If you do not know how to include it, you can check by clicking here.
To get to know about the LED pattern let’s start by writing a mini instruction.
LDI R17, 0XFF // 0b11111111
First, we took a general-purpose register R17, and loaded the hex FF value in it. Here hex value FF means 8 ones in binary.
OUT DDRD, R17 // config as output
Also, keep in mind that to configure any port for input or output we use a data direction register. Here we need the data direction register for port D so we gave the value of R17 to port D.
LOOP:
LDI R16, 0X01 // r16=0b00000001
OUT PORTD, R16 // port d= 0x01
Here in the label loop, we loaded 1 in the general purpose register R16. And then we output that 1 on port D which means that only LED is on and all others are off.
LDI R16, (1<<1) //r16=0b00000010
OUT PORTD, R16
Here the value on the right side of the bracket means pin number and the left side value means the which we want to give. This means that we shift the value 1 on the pin number 1.
LDI R16, (1<<2) //r16=0b00000100
OUT PORTD, R16
Here we shift the value of 1 to pin number 2.
LDI R16, (1<<3) //r16=0b00001000
OUT PORTD, R16
Here we shift the value of 1 to pin number 3.
LDI R16, (1<<4) //r16=0b00010000
OUT PORTD, R16
Now we shift the value of 1 to pin number 4.
LDI R16, (1<<5) //r16=0b00100000
OUT PORTD, R16
Here we shift the value of 1 to pin number 5.
LDI R16, (1<<6) //r16=0b01000000
OUT PORTD, R16
Now we shift the value of 1 to pin number 6.
Call DelayMEGA //function used for delay
LDI R16, (1<<7)
OUT PORTD, R16
Call DelayMEGA
JMP LOOP
DEBUG:
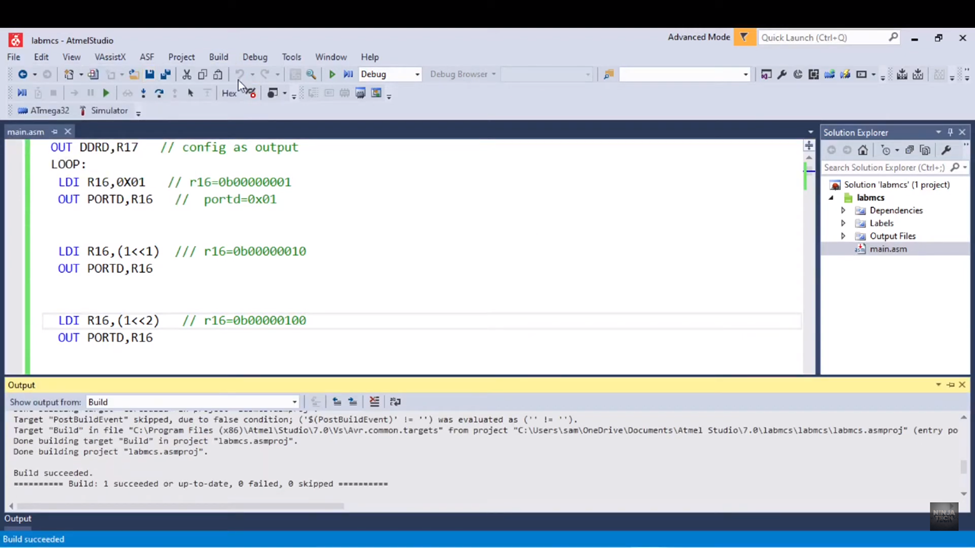
You can see below FF value has been stored in R17, and all the pins have been configured as output.
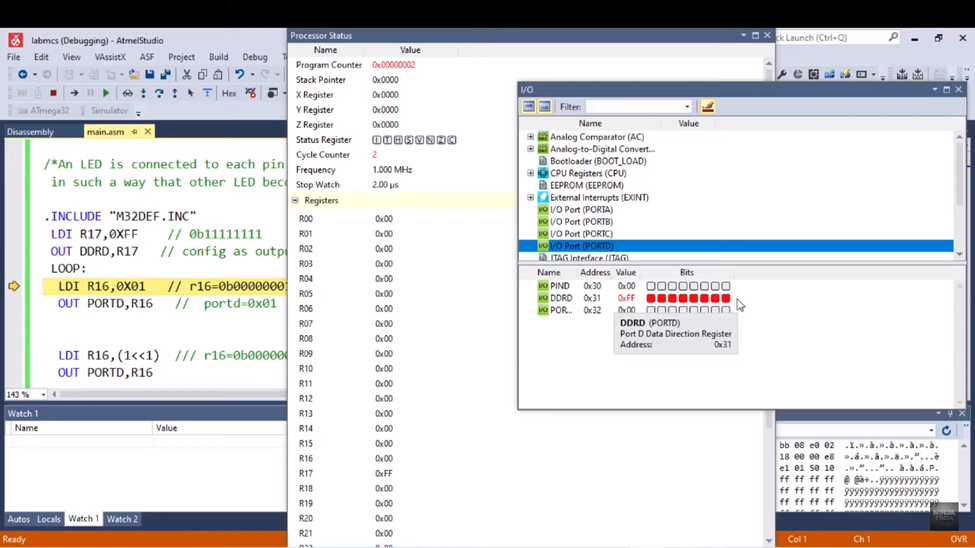
Below you can see R16 has the value 1 and first LED has the 1 value and it is glowing.
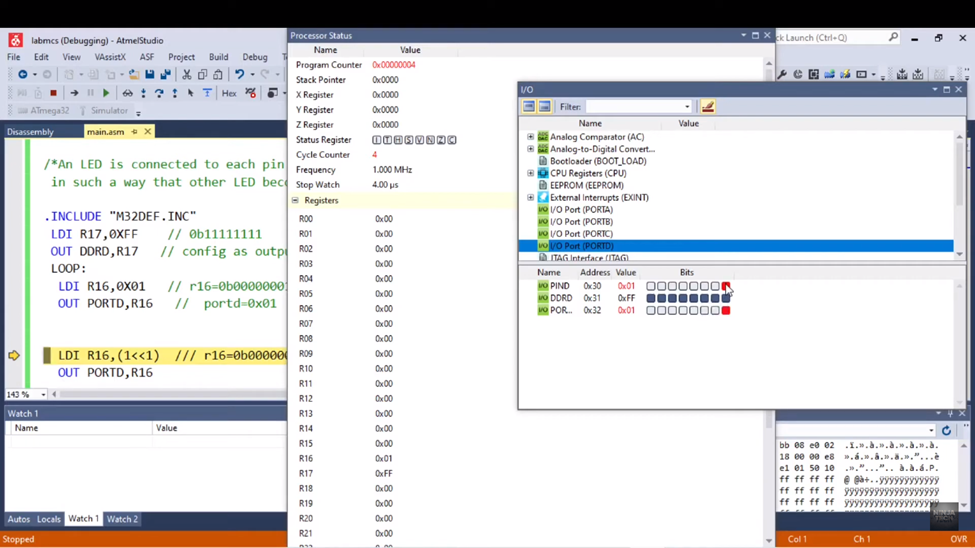
Below you can see R16 has the value 2 and first LED has the 1 value and it is glowing.
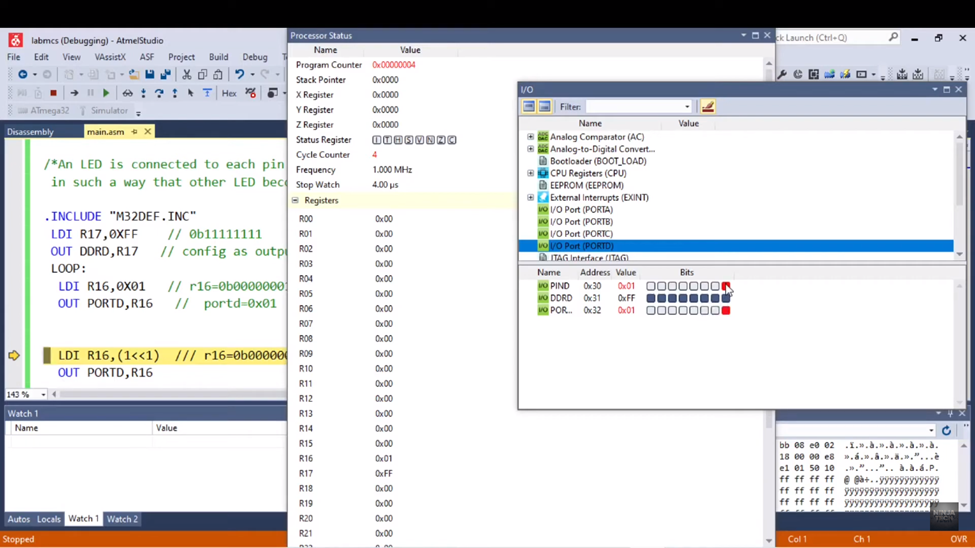
Similarly, the same pattern goes on for the remaining LEDs.
Let’s analyze our results through the proteus and you can clearly see at one time only one LED is on and all others are off.
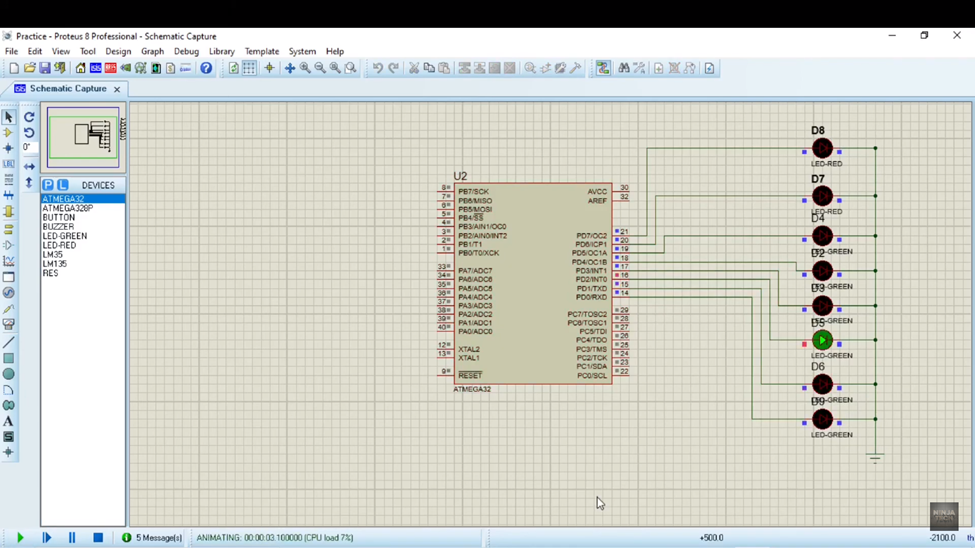
Conclusion
In conclusion, utilizing the Atmel Studio Assembly language to create an LED pattern and then recreating it in Proteus is a satisfying and instructive project. Through this project, we will be able to learn more about the details of low-level programming and the functions of microcontrollers. The LED pattern in the Proteus simulation serves as a visual representation of our work as we write assembly code and work with registers.
In addition to improving our programming abilities, this practical experience offers insightful knowledge about the useful applications of microcontrollers. In summary, this assembly language project is a rewarding experience that connects theory to practical simulation for learning LED patterns on Atmega32.
For Complete Trial Watch the Video: LED PATTERN in Atmega32 using ATMEL STUDIO Assembly & PROTEUS
For more blogs explore the website: https://ninjatech.live/